[jQuery] AJAX로 서버에 다양한 데이터 주고 받기
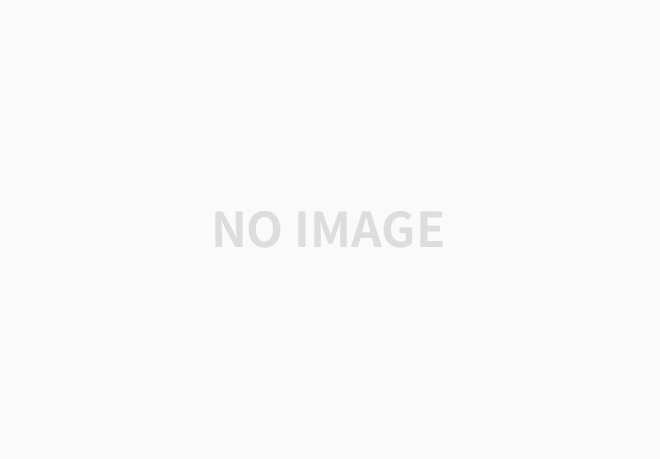
웹 페이지, 웹 어플리케이션(Client)은 특정 데이터를 가지고 서버에 데이터를 요청하면
서버는 그에 응당하는 데이터를 클라이언트에게 전송한다.
이번 포스트에서 다뤄볼 주제는 데이터를 서버에 요청하고
객체 타입의 데이터들을 비동기방식으로 클라이언트에 전송하는 것이다.
특정 데이터를 가지고 서버에 데이터 요청하고 응답받기
1. String 데이터를 응답받기
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.1/jquery.min.js"></script>
<body>
<p id="demo"></p>
<button>클릭</button>
<script type="text/javascript">
$(document).ready(()=>{
$("button").on("click", function() {
$.ajax({
url:"./hello",
type: "get",
data: {"id" : "abc", "pw" : "123},
success: function(data){
console.log(data);
$("#demo").text(data);
},
error: function(){
console.log("error");
}
}) // ajax
})
})
위의 소스코드는 id는 "abc"이고, pw는 "123" 인 data를 가지고
./hello에 있는 데이터를 요청하는 소스코드라고 볼 수 있다.
참고로 url에 ./hello라고 입력하면 매핑 시켜주는 web servlet이 다음과 같이 존재해야 한다.
@WebServlet("/hello")
public class HelloServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("service() 실행");
String id = req.getParameter("id");
String pw = req.getParameter("pw");
System.out.println("id: " + id + " pw: " + pw);
// String
String str = "안녕하세요";
JSONObject jObj = new JSONObject();
jObj.put("str", str);
resp.setContentType("applicatioin/x-json; charset=utf-8");
resp.getWriter().print(jObj);
}
Client에서 request 할 때, 서버에 넘겨준 data를 getParameter()로 받아 각각 변수에 저장하였다.
콘솔에 두 변수를 출력해보면 다음과 같이 잘 출력되는 것을 확인할 수 있다.
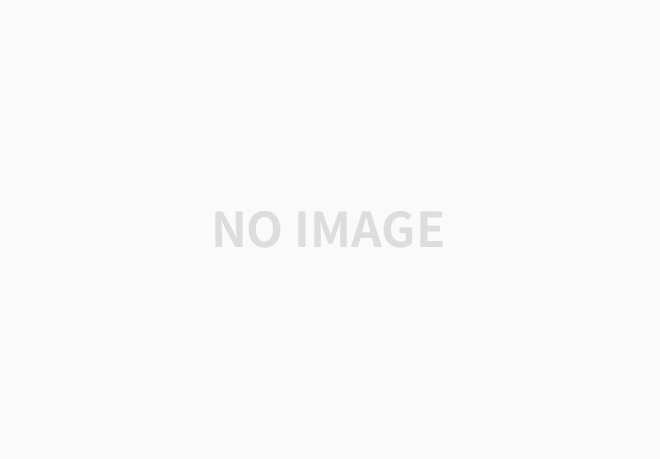
그 후에, JSONObject 클래스의 put()(HashMap의 put()과 동일)를 이용해 키와 값을 설정한 뒤
print()의 인자로 변수 jObj를 넣어주면 서버에서는 jObj 데이터를 클라이언트에게 전송할 것이다.
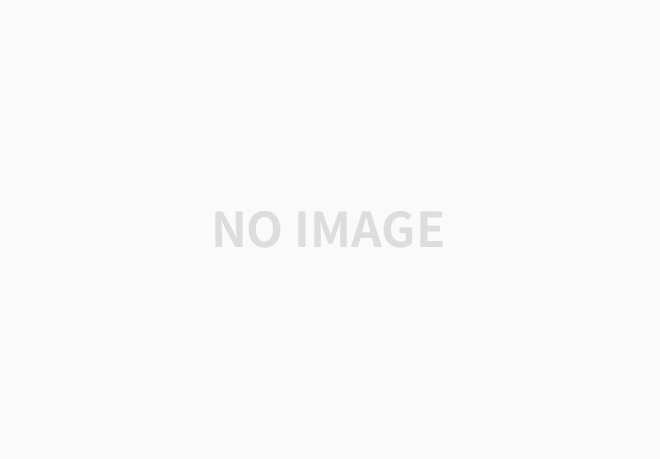
다음과 같이 Object 타입으로 데이터를 잘 전달받은 것을 확인할 수 있다.
"안녕하세요" 라는 문자열을 <p> 태그에 출력하기 위해서는 data.str이라고 입력하면 되겠다.
success: function(data){
console.log(data);
$("#demo").text(data.str);
},
2. 객체 데이터를 응답받기
먼저, 다음과 같은 Human 클래스가 있다고 가정하자.
package dt;
import java.io.Serializable;
public class Human implements Serializable {
private String name;
private int age;
public Human() {
}
public Human(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "Human [name=" + name + ", age=" + age + ", getClass()=" + getClass() + ", hashCode()=" + hashCode()
+ ", toString()=" + super.toString() + "]";
}
}
Human 인스턴스를 생성하고, 클라이언트에 전송해보자.
Human human = new Human("홍길동",24);
JSONObject jObj = new JSONObject();
jObj.put("human", human);
resp.setContentType("applicatioin/x-json; charset=utf-8");
resp.getWriter().print(jObj);
jObj 변수에는 human 이라는 객체가 저장되어 있다.
이 객체를 클라이언트에 전송하는 방법은 위와 동일하다.
다만, 인스턴스 변수의 모든 값을 확인하려면 JSON.stringify()를 이용하면 된다.
console.log(JSON.stringify(data));
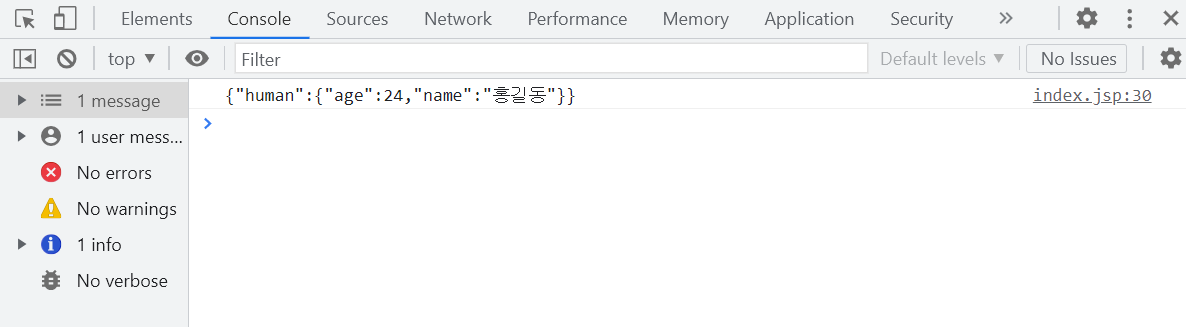
3. list 데이터를 응답받기
List<Human> list = new ArrayList<>();
list.add(new Human("홍길동", 24));
list.add(new Human("성춘향", 16));
list.add(new Human("홍두깨", 22));
JSONObject jObj = new JSONObject();
jObj.put("list", list);
resp.setContentType("applicatioin/x-json; charset=utf-8");
resp.getWriter().print(jObj);
list에는 총 3개의 객체가 들어있다.
응답받은 list를 stringify() 한 다음, console.log()로 출력하면 다음과 같은 결과를 얻을 수 있다.

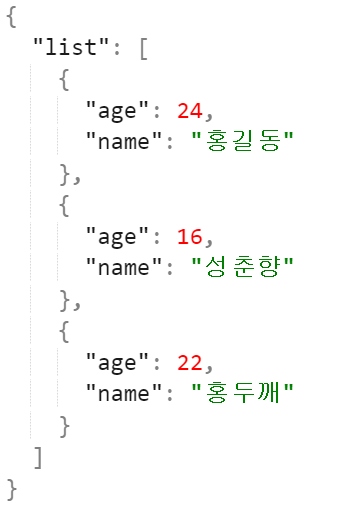
list[n] 이라고 입력하면 list에 포함 돼있는 객체 중, n번째 인덱스에 있는 객체에 접근할 수 있다.